pyqtgraphをPyQt5環境からPyQt6環境に移行する際のDeprecationWarning: GraphicsWindow is deprecated, use GraphicsLayoutWidget insteadの対処方法を説明する。
結論
PyQt5:GraphicsWindow()
PyQt6:GraphicsLayoutWidget(show=True)
コード例
下記のグラフ表示プログラムを例に説明する。
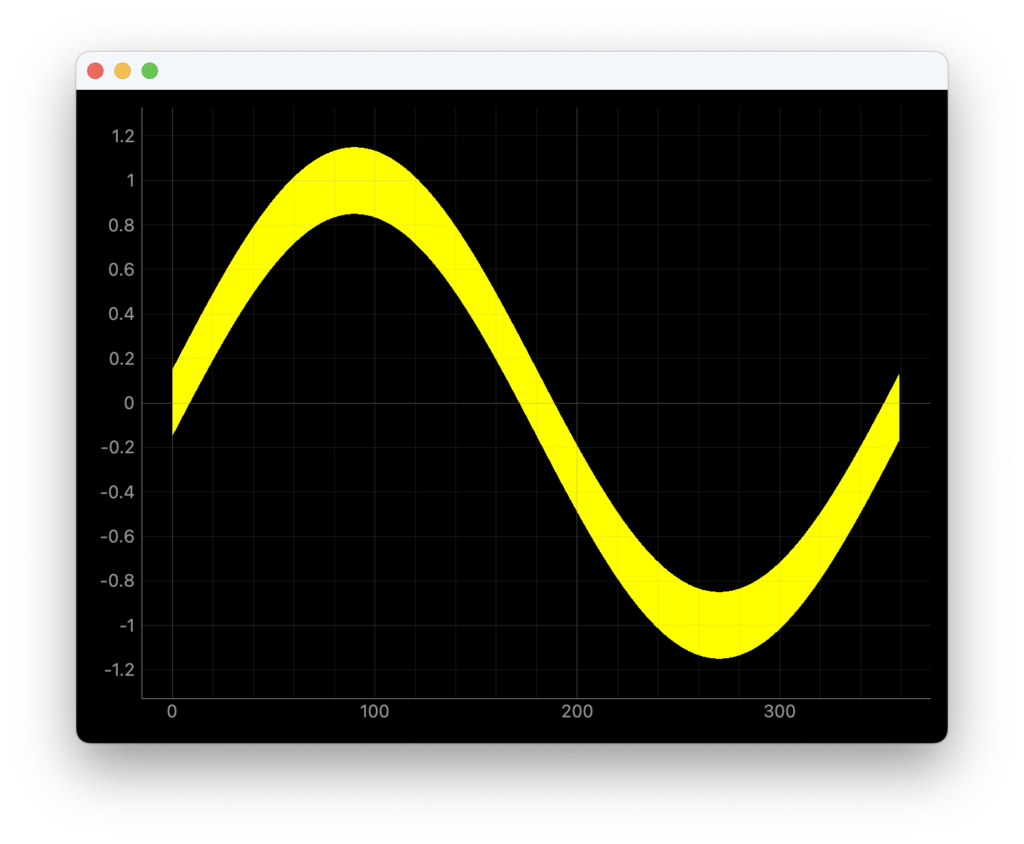
PyQt5のコード
#!/usr/bin/env python3
import sys
import numpy as np
from PyQt5.QtGui import QPen, QColor
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5 import QtCore
import pyqtgraph as pg
class GuiWindow(QWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.setGeometry(0, 0, 500, 45)
self.show()
self.make_xy_data()
self.plot()
def make_xy_data(self):
self.x = range(0, 360)
self.y = np.zeros(360)
for i in range(360):
self.y[i] = np.sin(2 * np.pi * i/360)
def plot(self):
pen = QPen(QtCore.Qt.yellow, 0.3)
self.graph = pg.GraphicsWindow() # ?
self.graph.move(11, 93)
self.p = self.graph.addPlot()
self.p.plot(x=self.x, y=self.y, pen=pen)
self.p.showGrid(x=True, y=True)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = GuiWindow()
sys.exit(app.exec())
PyQt6のコード
#!/usr/bin/env python3
import sys
import numpy as np
from PyQt6.QtGui import QPen, QColor
from PyQt6.QtWidgets import QApplication, QWidget
import pyqtgraph as pg
class GuiWindow(QWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.setGeometry(0, 0, 500, 45)
self.show()
self.make_xy_data()
self.plot()
def make_xy_data(self):
self.x = range(0, 360)
self.y = np.zeros(360)
for i in range(360):
self.y[i] = np.sin(2 * np.pi * i/360)
def plot(self):
pen = QPen(QColor(255, 255, 0), 0.3)
self.graph = pg.GraphicsLayoutWidget(show=True) # ?
self.graph.move(11, 93)
self.p = self.graph.addPlot()
self.p.plot(x=self.x, y=self.y, pen=pen)
self.p.showGrid(x=True, y=True)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = GuiWindow()
sys.exit(app.exec())
差分
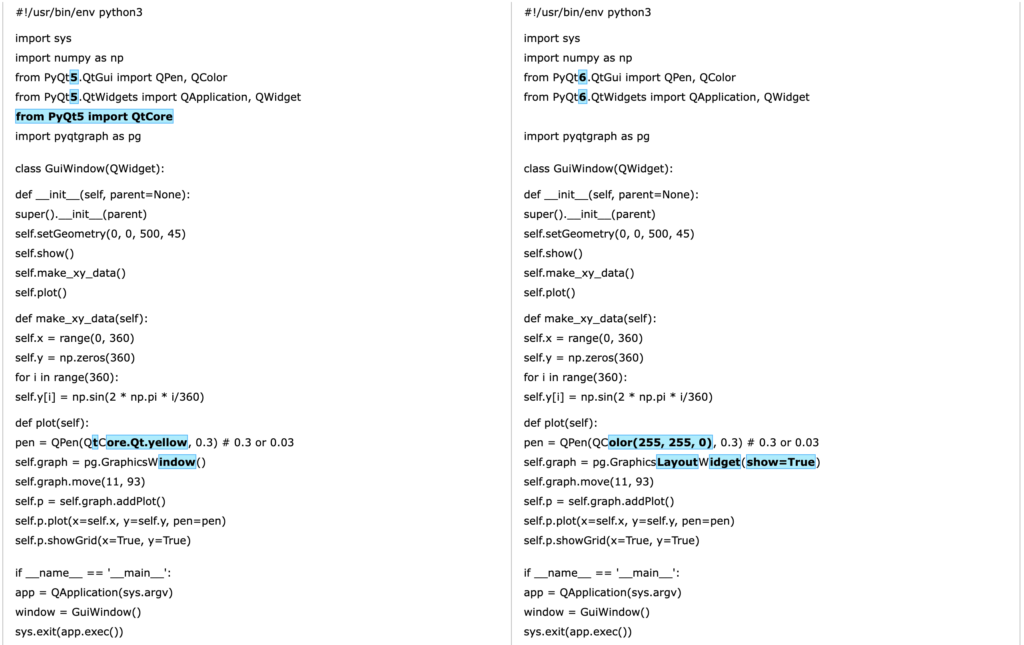
その他、PyQt5からPyQt6への移行方法の基本は下記記事を参照。
まとめ
GraphicsWindow is deprecated, use GraphicsLayoutWidget insteadの対処方法を説明した。
コメント